Two CakePHP tricks
Posted on 24/8/08 by Felix Geisendörfer
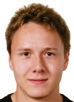
Hey folks,
here we go with post #5 of my 30 day challenge
Tip 1: Debugging frequently called functions
Imagine you got 100 assertions in the unit test of a function. Suddenly one or two unit tests fail. The returned result makes no sense whatsoever and you need to debug the function. If you just put a debug() statement in, you will see hundreds of outputs and would not know which one belongs to the case you are interested in. My favorite solution to this problem is to use the Configure class as a toggle for showing the debug information. But lets look at an example:
$r = Set::extract('/User[2]/id', $a);
$this->assertEqual($r, $expected);
// Test that is failing
$expected = array(4, 5);
Configure::write('debug', 1);
$r = Set::extract('/User[id>3]/id', $a);
Configure::write('debug', 0);
$this->assertEqual($r, $expected);
$expected = array(2, 3);
$r = Set::extract('/User[id>1][id<=3]/id', $a);
$t his->assertEqual($r, $expected);
// Function to debug
function extract() {
// do stuff
debug($valueOfInterest);
}
This makes sure you only get the debug output for the one particular case you are interested in.
Tip 2: Custom config file
This is a very simple one. To make your application configurable via a global config file, all you need to do is the following. Put this code inside your /app/config/bootstrap.php file:
This causes a file called /app/config/config.php to be included. You can of course use any other name, but I like this one. Created the file inside /app/config and then fill it with code like this:
'App.name' => 'Debuggable.com',
'App.guestAccount' => 'guest@debuggable.com',
'App.feedbackEmail' => 'feedback@debuggable.com',
'App.loginCookieLife' => '+1 year',
);
date_default_timezone_set('UTC');
Note: CakePHP expects an array named $config to be present in this file. So you could use App => array(name => val) to assign the sub values of the App key, however CakePHP also use the same key (App) for some of its settings. So you shouldn't completely overwrite it unless you know what you are doing. The keys used by CakePHP right now are: 'base', 'baseUrl', 'dir', 'webroot' and 'encoding'.
You can now globally access any of your config values. Here is an example inside a layout for setting the title element:
Alright, I hope you found something useful in the tips above,
-- Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
That's correct. The bootstrap file is loaded after the core config is loaded.
Very nice posts. Here at prayam.com we're considering Ruby on Rails against CakePHP for some bigger app and must say this config file tip deffo points on Cake, as the app is going to be deployed in many different variants :)
Thanks a lot, just used both just now. :)
@Matt: If you want to be deployed in multiple projects I'd say cake is the way to go anyway, simply because PHP is better supported.
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
I guess that the file /app/config/config.php will be loaded last, after the file core.php will load, right?