Google Analytics PHP Api (CakePHP Model)
Posted on 19/6/06 by Felix Geisendörfer
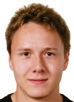
Deprecated post
The authors of this post have marked it as deprecated. This means the information displayed is most likely outdated, inaccurate, boring or a combination of all three.
Policy: We never delete deprecated posts, but they are not listed in our categories or show up in the search anymore.
Comments: You can continue to leave comments on this post, but please consult Google or our search first if you want to get an answer ; ).
IMPORTANT: The stuff found in this article is outdated and not working anymore. Please see: New Google Analytics API / DataSource! for a newer version!
Vote for this story on digg.com: Digg It!
I already talked about my CakePHP implementation of a Google Analytics API in some of my previous posts, and I also asked Google to clarify the legal status of such code. Almost a month has passed, and besides the initial we'll contact you about it again e-mail, I didn't hear anything from Google.
About 2 weeks ago, I recieved an e-mail in which one of my readers pointed out the existance of a couple wordpress plugins, that offer Google Analytics statistics. One of them also seems to be pretty popular and so far Google hasn't stopped any of the creators from providing them.
For this reason I decided to release my Api as well, and let you decide about the ways to use it. I'm sure you'll find some interestings things to do with it. Since I already started my new Web Model package over at cakeforge I just added the Google Analytics Model to it.
Online Demo
Want to see it working before you waste your time on it? Check out the demo I setup earlier!
How to Install
- In order to install the Api you need to get the latest version of CakePHP, and unpack it.
- Then download the Web Model and save it to app/web_model.php.
- After you've done that, download the Google Analytics Model and save it as app/models/google_analytics.php.
How to use it?
Here is a simple example how to do the authentication with Google Analytics and how to retrieve the executive overview report for the last 7 days from your first profile:
class AnalyticsController extends AppController
{
var $name = 'Analytics';
var $uses = array('GoogleAnalytics');
var $loginCookie = null;
function beforeFilter()
{
// Let's make sure we don't get php max execution timeouts
set_time_limit(60*5);
$email = 'my-account@gmail.com';
$pass = 'my-password';
$this->loginCookie = $this->GoogleAnalytics->login($email, $pass);
if ($this->loginCookie==GA_ERROR_ACCESS_DENIED)
{
trigger_error('Acces Denied', E_USER_WARNING);
}
elseif ($this->loginCookie==GA_ERROR_TIMEOUT)
{
trigger_error('Connection Timeout', E_USER_WARNING);
}
}
function afterFilter()
{
// Let's clean up the cache folder every 2 hours
$this->GoogleAnalytics->cleanUpCacheFolder('+2 hours');
}
function test_report()
{
$profiles = $this->GoogleAnalytics->listProfiles($this->loginCookie);
$firstProfile = $profiles[0]['id'];
$report = $this->GoogleAnalytics->getReport($this->loginCookie, $firstProfile, GA_REPORT_EXECUTIVE_OVERVIEW, '-7 days', 'yesterday', 10, GA_FORMAT_CUSTOM_ARRAY);
// Let's see how such a report array looks like:
debug($report);
}
}
If you want to find out about all functions and parameters that are supported, simply open up app/models/google_analytics.php and have a look at the source. Everything is documented fairly well and it should be easy to figure things out.
What you should know about the API
This Api essentially is a simulated browser that requests all data like a normal user would request them with a browser. That isn't neccessarly a problem, but if Google decided to change the interface for Google Analytics there could easily be propblems popping up.
In order to keep bandwidth usage low, most data (reports, logins, etc.) is cached for 2 hours by default, but only requests with the exact same parameters are beeing cached. The last parameter of most functions inside the GoogleAnalytics class is called $cacheExpires and can either be a timestamp for some point in the future, or a strtotime() compatible statement like "+30 minutes", this way you can specify the amount of time certain requests are beeing cached. Since Google only flows data in like every 6-24 hours you can easily set this value pretty high.
So I hope you enjoy playing around with this code, everything is licensed under MIT, so you don't have to worry about IP too much. I'd appriciate to get some feedback and will try my best to support those who struggle with the implemenation.
--Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
hi,
i am new with cake.
where do i save the file you have listed the source here for?
i would love to test this out, and give you feedback.
thanks.
rajendran.
india.
The source code I listed here would be a Controller that goes into app/controllers/analytics_controller.php.
For the other source code locations check out the installation instructions above.
I have made an incredible work! It could be very very usefull.
But I'm a MVC newbie. I install CakePHP correctly, I put your code in the correct files and paths, but I don't know how to call the application.
I try /GoogleAnalytics/ and all lowercase/singular combinations but I get a "Missing Controller" error or a "Cannot instantiate non-existent class: googleanalyticscontroller" error
What is wrong?
Sorry.. YOU have made an incredible work (I'm not english spoken)
Hi Humbert,
I'm glad you like my code ; ). Try to put a file called app/controllers/analytics_controller.php and paste the code from above in it (the AnalyticsController class). Then try calling your site via /analytics/testReport
I did it, and I even get a...
Fatal: Unable to load controller AnalyticsController
Fatal: Create Class:
in file : app\controllers\analytics_controller.php
..error. I us PHP5.1.1/MySQL4.1.7/Apache2 version and I have acos, aros, acos_aros and cake_sessions tables created in mysql.
Humbert: Without seeing the code I cannot really help you on this one. But I'm pretty sure it's to to your unfamilarity with CakePHP and the manual or #cakephp on freenode.net would be the best resource for that.
Sure, I understand you.
I will run it yes or yes :-) Then I will get you my feedback.
Thank you!
Hello, Thanks nice to see this code. Yet I have to test this one but hopes this will work.
My question is can we add the new site for google analytics using this API or it must to go manually to site and register the site.
Yogesh: This API assumes that you already have an Account at Google Analytics and setup the profile by yourself. It's main focus is to aggregate data from your analytics account, so that you can proccess it and display it in different places.
Hi felix, I tried to test the code, but I have a problem to see the results, all appear to be right, but the report is allways empty, I test it with different google analytics accounts, and nothing. Any suggestions?
Thank
[...] This example is from a report that scrapes Google Analytics and then presents the data. I wish I could say I was smart enough to figure out the Google Analytics stuff on my own, but I wasn’t. It’s a made-for-CakePHP solution and you can find it here at ThinkingPHP. Man, I love it when people smarter than me have figured stuff out. [...]
HI Felix,
This API is working perfectly. I got the Report from Google Analytics. Gr8 code.
Thank You
Hi Felix,
I have a client looking for EIS (Executive Information System) - basically a dashboard where they can see the overall health of the business. We need to be able to combine and then present the data not just from Google Analytics but also their financial information (e.g. recurring online subscription orders) but that's also online via their .net apps.
Any suggestions on a web based app that would possibly use your api or similar, and then also be able to take the financial data and present it in various key performance reports?
Maybe start with a reporting tool and work backwards?
I'm not sure where to start.
Am I asking too much?
Thanks,
Peter
Hey there...this is FANTASTIC. I've been dying for a GA API...however your demo seems busted and I'd really like to see it work. When I click exec overview on one of your profiles I get...
Raw Array Dump from Executive XML Report:
Access Denied
Hey JK: The Demo is working just fine. However I blocked access to all profiles but www.thinkingphp.org. So try this link: http://www.fg-webdesign.de/google-analytics/analytics/reports/executive/290712
Hope you'll be able to use the API for something cool ; ).
[...] Update: Here’s a hack-around of an API for Analytics if you want to start playing with some PHP. You can also bookmark this on del.icio.us or check the cosmos [...]
Yeah, the demo is busted for me...
Sorry, but you have no access to the Data on this Page!
Raw Array Dump from Executive XML Report:Access Denied
Jonathan: Please have the look at my comment above yours:
Hey JK: The Demo is working just fine. However I blocked access to all profiles but www.thinkingphp.org. So try this link: http://www.fg-webdesign.de/google-analytics/analytics/reports/executive/290712
Hope you’ll be able to use the API for something cool ; ).
Give this a try and let me know if you still have the problem ; ).
Felix: This is really great work. I've been able to get most everything I needed from your API. The one thing that I was not able to figure out from the google_analytics.php model file is whether or not you can set a parameter to have the results returned hourly (or monthly) instead of daily. As the Google Analytics UI allows you to "View By" Houry/Daily/Monthly, it seems like it should be possible. Do you know if there is a way? Did I miss something?
Thanks again,
Jason
Hey Jason,
hm I totally forgot about this option when creating the Model. Now that you mention it I just checked if reports like this can be extracted from GA as well and it turns out they can. So it's probably one of the parameters in the report url that I didn't figure out and left empty. Right now I'm lacking the time to implement this, but if you feel like doing so I'd highly appreciate it. Shouldn't take longer then an hour I guess.
Felix:
Thanks for the response. I did try entering different values for the other parameters, but it never seemed to change the results (or I got an invalid response). I'll keep trying - if I figure it out, I'll post it back here. If you happen to have time to figure it out first, please let us know.
Thanks again. Happy New Year.
Jason
[...] ThinkingPHP and beyond » Google Analytics PHP Api (CakePHP Model) Google Analytics pro cakephp (tags: cakephp google analytics) [...]
Nice work!
Felix -- awesome effort, hats off to you.
cheers
J.
[...] There doesn’t seem to be a Google Analytics API EXCEPT people have found a way to do it, see Felix Geisendörfer’s great work in Cake PHP (a popular PHP MVC framework). [...]
Hi Felix,
Can you help me...
How do you get this:
http://www.fg-webdesign.de/google-analytics/analytics/cron
From the raw array...
Can you give me the php code which is behind this:
http://www.fg-webdesign.de/google-analytics/analytics/cron
I'm sure you'll help a lot of newbies... and you'll open your post to a larger audience !
Thanks by advance,
Jeanviet
I finally succeed !!!
Here's the result:
http://jeanviet.info/cake/index.php/analytics/stats
Would it be possible to use this API without CakePHP? How much work would be involved in making a standalone API? What aspects of CakePHP do you rely most heavily on?
[...] Google Analytics API。厄,竟然不存在。但是有人已经用模拟用户提交的方式,变相实现了部分API。 [...]
Hey Jeanviet,
Can you give me the php code which is behind this:
http://jeanviet.info/cake/index.php/analytics/stats
I installed the CakePHP and the default page comes up as,
CakePHP Rapid Development
Your database configuration file is present.
Cake is able to connect to the database.
Now how I can get the google-analytics data in this?
Could you please give me a sample script?
Thanks!
Shashi
Hi Hi Felix,
I have installed CakePHP inside the Apache root and I made Apache DocumentRoot to point to CakePHP webroot. Now the url http://localhost/ gives me the default Cake page, which clearly suggest that Cake is configured properly.
As i'm bit new to Cake and I don't know how to run/invoke sample scripts, like the one above(Google Analytic). I followed the same above steps, but no luck.
-----------------------------------------------------------------------------
These are the steps which I followed.
-Placed web_model.php in cake/app folder,
-analytics_controller.php in cake/app/models folder.
-Placed the above sample authentication script Google Analytics in a file Analytics.php in the Cake webroot. But when I tried to invoke the file Analytics.php using the URL http://localhost/Analytics.php I got the error
“Fatal error: Class ‘AppController’ not found in C:\wamp\www\cake\app\webroot\Analytics.php on line 2.
What i'm doing is right? Please let me know where I went wrong!
Please help in running the above sample Google Analytics script.
Thanks in advance,
Twister.
Hi everyone!
I know it's a pretty old post but it still rocks and has success. I figure some of you have tryed to get data in hourly/monthly format as GA let's its possible, so the value to modify is the "dtc" one getting values '1' (for hourly), '2' (for dayly) and '3' (for monthly). I suggest you to create constant values as Felix does calling them for example:
//Timeline format
define('GA_TIMELINE_HOURS','1');
define('GA_TIMELINE_DAYS','2');
define('GA_TIMELINE_MONTHS','3');
and then modify the getReport method adding a new value ( I called it $timeline and inserted it between $format and $filter). Remenber to check it's not null and to modify in $vars array 'dtc' => $timeline
I hope it helps!
Hello,
Can any one address my previous query?
Is it possible to dump the Google Analtyic data to a dabatase using the above method?
If so please guide me. If possible with a demo script.
Thanks in advance,
Twister
Twister:
> -analytics_controller.php in cake/app/models folder.
Try to put it inside /app/controllers and see if that helps.
Shashi,
I hope it will help you...
http://jeanviet.free.fr/google/analytics.zip
More explications on what to do with this zip file (in french) here:
http://forum.jeanviet.info/comments.php?DiscussionID=66
Thanks a lot Felix for your work.
Hello!
Can anybody help with this... I didn't changed anything in thouse files, but it gives errors:
Notice: Undefined offset: 0 in /home2/wizards/public_html/cake/app/controllers/analytics_controller.php on line 75
Notice: Undefined variable: arrayReport in /home2/wizards/public_html/cake/app/models/google_analytics.php on line 415
When, I can see the statistic page, but it is just empty...
Thanks a lot for any comments...
Lana : Any indication of how you use the code would help. Put it in cake's bin right here and I'll take a look at it:
http://bin.cakephp.org/add/lana
Wow, it is working! Thank you a lot!!!
A few questions now...
1. I have few Google Analytics accounts by one e-mail and pass. Is it possible to choose, which account I would like to use?
2. Now it's takin data just from Executive Overview view. Is it possible to take data, for example, from Marketing Summary or other views?
Hello,
I've put my "TestAnalyticsController extends AppController" in my app/controllers folder but there's no AppController there. What's wrong?
Thanks for any replies.
Spule: If you need a custom AppController class then put it in /app/app_controller.php. If you don't, then CakePHP uses an empty default one located somewhere in the /cake folder. Anyway, you might want to read a little through the manual http://manual.cakephp.org/ if this is your first time in the kitchen, all of this should be covered there in more depth ; ).
Good luck with your project, Felix
Lana: #1: Yes, just run the login() function again before using a different account during the same request.
#2: Yes, in the example above I use the parameter GA_REPORT_EXECUTIVE_OVERVIEW. Check the GoogleAnalaytics model and you'll find all the other views defined as constants in there as well.
Felix Geisendörfer: I don't need any customized app controller; I am just trying the above example and it isn't able to find the AppController class. When I remove the "extends AppController" directive, it says it can't find GoogleAnalytics either.
I have PHP 4.3.6 with safe mode.
Spule:
> I have PHP 4.3.6
This is seriously old and before trying anything else you should upgrade.
How do you call the controller? I still think you might want to read about CakePHP itself a little more.
I don't know whether it's a correct way, but I do it thisway:
require('cakephp/app/controllers/GATestController.php');
$test = new TestAnalyticsController();
$test->beforeFilter();
$test->testReport();
$test->afterFilter();
Špule: Yeah it's really not. You should be calling your controller via your local webserver using something like:
http://localhost/cakephp/analytics/testReport
Your controller should be named. AnalyticsController and saved in /app/controllers/analytics_controller.php. But really, you got to learn the basics of CakePHP before really using this Model in a project. Can't really walk you through this myself, but we have wonderful community that you can talk to in #cakephp on freenode.net and on the mailing list. And again: Take a look at the manual, you'll not regret it.
Felix,
Fantastic work. The whole thing is very easy to implement as well.
I actually coupled the views with PHP/SWF charts (http://maani.us) to give the 'Google' look.
One think I have noticed :
The 'GA_REPORT_ABSOLUTE_UNIQUE_VISITORS' when called through the getReport() seems to create an error (notice error) since the last array as the index name empty (see below).
While I was too lazy to modify the model, adding error_reporting(0); to my controler just shut it up (please do not follow that example .. bad, bad coding).
Array
(
[0] => Array
(
[Name] => Absolute Unique Visitors
[Items] => Array
(
[0] => Array
(
[] => 159 // [] is empty Model xmlReportToArray () does not like it.
Also, I am sure you have other projects, but it would be nice to have this in a repository open source. I have added some functions and currently creating a MVC for Google Base. It would be nice to have a living project .. just a thought.
Thank you for sharing your work, it was greatly appreciated.
Felix: Thank you, now I see that I should really read the manual in detail.
Michel: Cool to hear that you are having some fun with this class. A little tip about shutting up PHP notices and warnings: simply put an '@' before the function call that is producing it and all output from this function (even echo's I believe) will be suppressed. Quick and dirty, but comes in handy sometimes when used responsibly *g*. About moving this to an open source repository: I currently am finishing up some groundwork for bringing HTTP web services to CakePHP natively. Google Analytics is most likely on of the first things I'm going to tackle again, and it'll become much nicer to use. After that I'll see if there are still things missing and if people would feel like contributing to it on a regular basis.
Špule: Np, I know the feeling of wanting to get something to work instantly, but hey it shouldn't take you all that long to pick the things up from the manual that you need ; ).
What about Sam Minnee comment?
Would it be possible to use this API without CakePHP?
ancient / Sam Minee:
I mostly used CakePHP for caching and might have also used one or two of it's convenience functions for standard php stuff (e.g. am instead of array_merge).
A skilled coder should be able to decouple the class from the framework in < 1 hour, but in case you are starting a new project I would highly recommend you to save that time and invest it in learning CakePHP instead. HTH
Hi,
Looking forward to using this code. Looks nice so far. Little problem though. I have a brand new functioning install of cake on a macbook pro. I've gotten to the point of trying your demo code and am stonewalled by the message:
Missing Method in AnalyticsController
You are seeing this error because the action index is not defined in controller AnalyticsController
For what should I check, exactly?
michael: Ok, I update the code above. Try the following now:
http://localhost/cake-install-dir/analytics/test_report
That should work. Let me know if it doesn't. However you might also want to consider having a look into the cake manual over at http://manual.cakephp.org/ if you haven't done this already.
-- Felix
Is there a way to combine Webmaster Central Tools with Google Analytics information using your API? I think it would be great to mix some reports for example the search query info with the visits.
Thanks
Alex C: No idea what "Webmaster Central Tools" is but my guess is that everything that is accessible in one way or the other can also be mashed up with anything else.
I used the CakePHP Google analytics API referenced below and got great results. I can get reports on whatever I could from the Google Analytics page, but it is faster and I can setup a crontab task to email reports daily to my boss with things like who looked at the join now page (just send the GoogleAnalytics->runReport function a regular expression of (^/join_now/$)|(^/join_now/\?from.*), which gives me all of the pages I know that uniquely report when a person comes to the join now page on our site).
I recommend anyone needing data from google just install cakephp in a subdirectory of their site (or on the devserver as I did - behind a firewall so only works from inside office) and then follow the instructions at the link Mislav gave below.
Seriouslly, this API that was shared with the world by a lovely developer has saved me from having to switch analytics companies for lack of emailed reports that can be customized. We needed the report to have things from our database, so an inhouse solution using this API was exactly what we needed. Here are some functions that might help if you add them as methods to the GoogleAnalytics class that I created:
/*
Add this function to analytics_controller.php
parsePageReport($report,$col='') - for the given report, returns the cumulative sum of all items returned from GA_REPORT_TOP_CONTENT report.
ex:
$n=7;//last 7 days
$rep = $this->GoogleAnalytics->getReport($this->loginCookie, $profileId, GA_REPORT_TOP_CONTENT, "-$n days", 'yesterday', 10, GA_FORMAT_CUSTOM_ARRAY,getRegExpr($keywords));
returns: nothing, modifies report to contain either a number or array, depending on whether $col is present. If $col is present (i.e. $col="Uniq. Views"), returns the sum of the columns over all items in the report
*/
function parsePageReport(&$report,$col=''){
$keys = array_keys($report[0]['Items'][0]);//Get array keys if any data returned from report
$res = array();
//numItems = count($report[0]); this can be used if an average and not a total is desired
foreach($report[0]['Items'] as $item){
foreach($keys as $key)
$res[$key] += $item[$key];//Incrementally add to the total
}
//If they requested only a column be modified, set the $report as being the value found in the sum of this $col
if($col != '') $report = $res[$col];
else $report = $res;
}
/*
Add the following functions outside of the GoogleAnalytics.php class for global availability
Example Argument:
$stringArray = formated array that contains search terms in the first column and true/false argument in second to wheter or not the string to be found should terminate or allow for wild characters at the end
$strings= array(
"Tour" => array( array('/tour/',false),array('/tour/?from',true)),
"Join Page" => array( array('/join_now/',false),array('/join_now/?from',true))
)
$regExp = getRegExpr($string);
*/
function getRegExpr($stringArray=''){
$reg='';
array_walk_recursive ($stringArray,'replaceSpChars');//Clean special characters
foreach($stringArray as $st)
$reg .= "(^{$st[0]}". ($st[1] ? ".*" : '$') . ')|';
$reg = rtrim($reg,'|');
return $reg;
}
function replaceSpChars(&$st,$key){
$specialChars = str_split('.*+?-|^$');
$toRet = $st;
foreach($specialChars as $char){
$toRet = str_replace($char,"\\$char",$toRet);
}
$st = $toRet;
}
Hope this helps someone, and helps everyone to see that the Google Analytics API for CakePHP is very usable and mature, a must for all developers who need to leverage their Google analytics accounts for their full worth!
Thanks for your reply, Felix.
Sadly, I am now getting the following error instead:
Missing Method in AnalyticsController
You are seeing this error because the action test_report is not defined in controller AnalyticsController
Obviously it is; I'm using your sample file.
Any ideas about what to try or to look for?
Thanks!
Michael
duh. Now I see I am replicating your original typo! Sorry, I fixed that and it works! Thanks a lot.
Hi~ Felix...
I tested,
(http://localhost/cake-install-dir/analytics/test_report)
but there is an error like this
"Fatal error: Call to undefined function: curl_init() in c:\apm_setup\htdocs\cake\app\web_model.php on line 46"
How can I solve this?
Thanks for your reply~
Michel, any chance you would make a tutorial for integrating PHP/SWF charts and the GA API? I've seen one explanation of using PHP/SWF with cake but I'm finding the leap from that to using it for GA pretty daunting.
Thanks.
Michael
Darkrimer, I think that means you need to recompile php with curl support (or in your case, add it to php.ini). Is curl listed in the output of phpinfo?
We were using Webalizer Version 2.01 for website stats. But I have learnt much about Google Analytics lets try this also. Good Info here.
Great work! Been for looking for something like this for ages!
2 questions though:
1. Can I use this to stitch together seperate but related reports from the Analytics system? For example, I would like to have campaigns and ad groups in the same file.
2. Not sure if this is necessary, but will you be updating your code to support the new Analytics interface?
Also, I have multiple linked accounts, how do I access a profile from another linked account?
trnsfrmr:
#1: Yes you can. Download both reports into an array and then merge those arrays to your liking.
#2: Yes, I will eventually update it and release a new version that will be easier to use and more powerful.
> Also, I have multiple linked accounts, how do I access a profile from another linked account?
Not sure, haven't tried that out so far.
Does this work on the newest update that just came out? I could not get your link to work for me.
Dave: Yes it does still work. See: http://www.fg-webdesign.de/google-analytics/analytics/reports/executive/290712.
I will however release a version that makes use of the new interface at some point.
Hey Guys,
this is a very nice tool felix, thanks a lot!
Unfortunately I have completely no experiences in Cake. I tried to read your code in order to implement your API for my purpose - but did not get any further after hours. Perhaps someone could help me?
I want to read out from "Content / Top Content" all result URLs filtered for the string "raumfahrer.net/news".
Actually I don't know how to get to this submenu Top Content and how to work with the filter argument of getReport().
Thank you for any help. :-)
pikarl
Can someone please help me write a phpCake wrapper for http://teethgrinder.co.uk/open-flash-chart/ ?? It must be pretty easy because there is already a PHP wrapper which is OO. Open flash chart is also open source - GPL.
monk.e.boy
pikarl: Sorry, but this kind of support is out of reach for me right now : /. Not to soon from now I'll release a new version of my Google Analytics API that will have more comprehensive instructions and should be easier to use. Meanwhile I can only suggest you to checkout CakePHP as you certainly won't regret it.
monk.e.boy: Sorry, nobody will do your work around here : /. At least not if you ask in such a general manner in the wrong place (this is a blog post about google analytics, not about charting technologies). Maybe try the mailing list or hop on IRC.
What could I be doing wronh? I am getting this error message
Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at C:\Software\Apache2.2\htdocs\cake\app\models\google_analytics.php:101) in C:\Software\Apache2.2\htdocs\cake\cake\libs\session.php on line 154
Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at C:\Software\Apache2.2\htdocs\cake\app\models\google_analytics.php:101) in C:\Software\Apache2.2\htdocs\cake\cake\libs\session.php on line 154
Warning: Cannot modify header information - headers already sent by (output started at C:\Software\Apache2.2\htdocs\cake\app\models\google_analytics.php:101) in C:\Software\Apache2.2\htdocs\cake\cake\libs\session.php on line 155
CakePHP Rapid Development
Missing Model
No class found for the GoogleAnalytics model
Notice: If you want to customize this error message, create app\views/errors/missing_model.thtml.
Fatal: Create the class below in file : app\models\google_analytics.php
Ignore the last message - solved the problem. It works fine - thanks for the great work.
this only works for one account,
i.e. if your user is attached to many accounts it will only show the default
scid refers to the account.
ACCOUNT
sorry
GB
dang
-select id="account" name="scid" onchange="this.form.submit()"-
-option value="xxxxxxx" selected=""-ACCOUNT -/option-
-/select-
with google analytics update (someone know how to see hourly reports in new version ?) you tip doesn't work anymore
Hi Felix.
Have you done an API version that support new G Analytics interface?
Hi There. I have been using the Analytics Api successfully for 6 months, but beginning July 23rd, 2007 $this->GoogleAnalytics->getReport(…) returns an empty string. As you may know, google recently updated their user interface, and as a result they published that the old interface would not be available after July 17th, 2007.
Felix, would you pretty please look into this. I am digging through the code as well, and will let you know if I figure out where the data parsing stops working, as I know that your API relies on the Analytics UI and the pages that were available in the previous version.
I may be wrong, but is there anyone else? Will keep you up to date if I find a solution.
Felix we need an update
Hey folks: I'm working on a new version right now. It will most likely not be compatible with the old one. This has two reasons: It will be better and the API more comfortable to use. And on the other site it seems like the reports returned by the new interface have a different format then the old ones. I'll do a new post and update this one when there will be a release - stay tuned.
Sorry for the inconvenience, I hoped Google would not disable the old interface that soon.
Thanks for the update. Could you give us a ETA?
Phil: Not before Sunday. My main problem right now is that I need to get Cake's new HttpSocket to work with HTTPS. Once that is solved things should evolve quickly.
Thanks Felix, we appreciate the hard work you've done to bring this API back to life, look forward to seeing the new version :)
Chad / everybody: Quick update:
I am currently unable to do any dev work as I'm in Berlin and struggling to get my new laptop (macbook) setup as a dev environment : (. Once that is done I'll try to pickup API development ASAP again. Sry for the delay.
Thanks for the update! I want to get a macbook pro too soon, let me know how you like it :)
Just tried looking at your demo for Google Analytics. The following error came up when clicking on thinkingphp.org
Notice: Undefined variable: report in /www/htdocs/w0065c6b/google-analytics/app/views/analytics/reports/default.thtml on line 2
Jenifer: Yeah the API is broken right now due to Google being evil, err changing their service interface : /. I'm working on a fix but things have not progressed as I hoped so far.
Bummer, hope you get it fixed soon, would love to start using it.
Analytics is slowly allowing AdSense integration for tracking though, so that's a plus. hopefully they will release an official API sooner or later.
I wonder if someone could help me here, i'm new to PHP and have basic knowledge of Cake PHP.
I've just unpacked Cake etc and it all appears ok, except i don't want to setup the MySQL side of things as it might mess up my current system. Will this affect the Google Analytics API?
When i try and access http://www.thepricesite.co.uk/Cake/app/models/google_analytics.php
I get the below message:-
Warning: main(APPweb_model.php): failed to open stream: No such file or directory in /home/t/h/thepricesite_co_uk/Cake/app/models/google_analytics.php on line 14
Fatal error: main(): Failed opening required 'APPweb_model.php' (include_path='.:/usr/local/lib/php/') in /home/t/h/thepricesite_co_uk/Cake/app/models/google_analytics.php on line 14
Any ideas? Help!
Michael
Hi, I posted some code here yesterday to access GA2 data. I don't see it, so don't know if it's a technical glitch or waiting for approval. If this shows, but not the earlier, I'll re-post.
-David
Tried again, but it didn't post. Maybe too long (146 lines)?
Sorry-
-David
dlh: Please use cake bin: http://bin.cakephp.org/add/dlh
OK, Felix, did that. After I hit Submit I got a message saying "The Paste is just temporary." Anything else for me to do? Thx.
dlh: Below the note field there is a checkbox that says 'Save'. Check it.
Then the url to your paste is the one you are redirected to after submitting it. Copy & paste that in here.
Like this?
http://bin.cakephp.org/view/2093657349
Thx-
-David
DLH: Thanks a lot for sharing.
Everybody: Please give his solution a try - I'm willing to accept patches for the current implementation once I know that they work for most of you and will merge them with my own code and credit you. However, I'll be gone on vacation for a week beginning Saturday, so if you get them in before that - that'd be great : ).
Unfortunately the new version of the API is delayed again as I got problems with SSH w/o curl.
-- Felix
PS: DLH: No need to apologize for modifying my code. It's published under the MIT license so you can do whatever it is you like to do with it besides removing the license : ).
Bummer, couldn't use the patch because I need to run specific results based on a filter string, but that argument was removed from getReport_dlh(...) in DLH's patch :(
I would pay for backward compatibility with my current controller if it could be done, would need the reports to come back in the same format as before as my XML parsing depends on it to sum the visitors to a specific page over a certain time period.
Boss has been riding me for a month, and I am just desperate to get analytics reports back so that I can cron them again and get him numbers.
Thanks guys, let me know after your vacation if you reach a solution, and we'll donate to this project since it is so vital to our current reporting solution.
Cheers- Chad
I know you read all these comments, but it is hard to read yours as I (and maybe some others here) are not familiar with the language the last post was in. If possible, would you mind translating your post into English for us?
Thanks :)
Chad
Hi, Chad, I'm just back from vacation and probably need a few days to catch my breath. I scrapped filters during my rewrite because I tend to bring in everything raw and then manipulate the results after they're in my database. (And, I was aiming for a quick & dirty fix. :) )
What GA reports are most important to you?
Best-
-David
I use GA_REPORT_ABSOLUTE_UNIQUE_VISITORS with a regex filter to get all pages in a certain category, and I vary the number of days that the report looks at.
And I parse the results with this function I wrote:
____________________
/*
parsePageReport($report,$col='') - for the given report, returns the cumulative sum of all items returned from GA_REPORT_TOP_CONTENT report.
ex:
$n=7;//last 7 days
$rep = $this->GoogleAnalytics->getReport($this->loginCookie, $profileId, GA_REPORT_ABSOLUTE_UNIQUE_VISITORS, "-$n days", 'yesterday', 10, GA_FORMAT_CUSTOM_ARRAY,$t->getRegExpr($keywords));
*/
function parsePageReport(&$report,$col=''){
$keys = array_keys($report[0]['Items'][0]);//Get array keys if any data returned from report
$res = array();
foreach($report[0]['Items'] as $item){
foreach($keys as $key)
$res[$key] += $item[$key];//Incrementally add to the total
}
//If they requested only a column be modified, set the $report as being the value found in the sum of this $col
if($col != '') $report = $res[$col];
else $report = $res;
}
_________________________________________
The regex function (which I call for the filter argument) just takes in a string and optional terminate, end string, etc. requirements and generates a regular expression that fits the arguments.
Thanks for your attention, and let me know if there is anything I can do to help.
-Chad
P.S. Hope your vac was great :)
DLH: I'm absolutely overwhelmed with things right now, so if you could help me to get this API back up and running (ideally with backwards compatibility) you'd not only make my and the day of many folks here, but I'd also make sure that your contribution gets the recognition it deserves in a blog post for it and a link to your site and stuff.
Of course I cannot expect you to do my work, so if you are busy yourself I'll try hard to get the new API out of the door soon, but meanwhile I'd appreciate any help to get the people who implemented it back up and running.
Thanks, Felix
OK, Folks, I've taken a crack at a Felix-compatible version of the getReport() method. No promises, needs testing, but let me know what you think.
http://bin.cakephp.org/view/204546619
Best-
-David
It works, but the xml->array is not parsing the xml properly. Comes up as a blank string rather than an array, as where if the format is xml then you can see properly formatted xml such as:
Absolute Unique Visitors
www.3hourdiet.com
August 12, 2007 - September 11, 2007
false
false
992
August 12, 2007
887
August 13, 2007
613
August 14, 2007
...
I'm gonna do some investigating on the xmltoarray right now, but good job. I didn't realize it, but the URL format for google analytics is actually not too scary, now that I have a better idea of how all of the functions work. Great work David, I hope to get this working 100% soon, and will post anything I find here as well
Shoot, it stripped the tags around the xml :( The above comment did contain xml tags around what are now just values
Chad, try cakebin: http://bin.cakephp.org/add/Chad
I'm running into some trouble with the reports that come back from google. Even if a date filter is applied (ie, say I just wanted yesterday's stats), it no longer returns just that day, but a whole week. The xml data returned is cluttered with all sorts of horizontalbar, group, etc. tags. Anybody had success on decoding what the structure of the reports are?
Hi,
I've the same error : "Undefined variable: arrayReport...' Can we have the solution you gave to lana here please?
Thanks,
Stephane
Still dont working?
Excellent work man.....you did'nt happen to write a .net class did you ;)
[...] read more | digg story [...]
[...] Google does not provide an official API to access Google Analytics data by external programs. Fortunately, programmers have developed unofficial APIs[ 1, 2] for doing the same, even though Google is remaining silent on the legality of using it. Google Analytics Reporting Suite is also based on an unofficial API developed by Nico, by studying the working of the web application for months. Fortunately, both Google and Adobe extended support to Nico for further developing the application. GARS is included in Adobe’s Showcase program and Google has offered assistance in making the program more secure. [...]
Offiziell gibt es keine Schnittstelle, um Daten direkt aus Google Analytics an ein Drittsystem zu liefern. Allerdings haben zwei Programmierer bereits letztes Jahr erste Schritte in diese Richtung unternommen und Prototypen vorgestellt. Auf Basis des PHP Frameworks CakePHP hat Felix eine einfache Google Analytics API entwickelt. Einen anderen Ansatz verfolgt Joe Tan mit seinem Wordpress Plugin für Google Analytics. Die derzeit wohl optisch beste Variante stammt von Joyce Babu: er nutzt das Adobe AIR Framework und stellt damit eine Desktop Suite zur Verfügung.
[...] sorry it took me forever, but after my old Google Analytics API fell apart due to the fact that Google published a new interface that also came with new reports / exporting formats I didn't have the time to come up with a new one. [...]
hi, can you help me?
why the code bellow respose nothing ?
-------------------------------------------------------------------
$content = "continue=http://www.google.com/analytics/home/?et=rese&hl=zh_CNt&service=analytics&nui=l&hl=zh_CN&GA3T=Che55Bdm7Gg&GALX=exmr15WRqoI&PersistentCookie=yes&Email=$myemail&Passwd=$mypass";
$address = gethostbyname('www.google.com');
$fp = fsockopen($address, 443, $errno, $errstr, 300);
if (!$fp) {
die("$errstr ($errno)");
return false;
} else {
$str = "POST /accounts/ServiceLoginBoxAuth HTTP/1.0\r\n";
$str .= "Host: ". $address ."\r\n";
$str .= "Content-type: application/x-www-form-urlencoded\r\n";
$str .= "Content-length: ".strlen($content)."\r\n";
$str .= "Connection: Close\r\n\r\n";
$str .= $content;
fwrite($fp, $str);
while (!feof($fp)) {
$line = fgets($fp, 2048);
echo $line;
}
fclose($fp);
}
-----------------------------------------------------------------------
[...] Auf Basis des PHP Frameworks CakePHP hat Felix eine einfache Google Analytics API entwickelt. Einen anderen Ansatz verfolgt Joe Tan mit seinem Wordpress Plugin für Google Analytics. Die derzeit wohl optisch beste Variante stammt von Joyce Babu: er nutzt das Adobe AIR Framework und stellt damit eine Desktop Suite zur Verfügung. KPI Newspaper Mit meinem Ansatz verfolge ich die wichtigsten Kennzahlen aus Google Analytics zu lesen und dann auf maximal einer DIN A4 Seite als PDF auszugeben. Hier ist ein Proof-of-concept, der zumindest schonmal Daten aus Google Analytics extrahiert. [...]
sir,
For Google Analytics api i have followed the steps written in http://www.thinkingphp.org/2006/06/19/google-analytics-php-api-cakephp-model/ as well as visited this url also http://www.thinkingphp.org/2007/12/18/new-google-analytics-api/
Please let me know which to follow and the required steps to implement the google analytics. Its important.
[...] Šeit var palīdzēt risinājums, kas prasa PHP prasmes un ir uzcepts pirms nepilna gada -Google Analytics PHP Api [...]
[...] currently they don't have an api Is there a Google Analytics API? but some guy has released some bits of code to interact with it ThinkingPHP and beyond Google Analytics PHP Api (CakePHP Model) __________________ my sites :irish jobs / seo faq / jobs in ireland / advertise jobs free / green card / skiing [1] [2] [3] [4][5] [...]
Hi,
can anybody help me.. Its urgent
I received the following error while running the script
Notice: Undefined variable: arrayReport in C:\Program Files\xampp\htdocs\cake1\app\models\google_analytics.php on line 416
Hi
I installed cakephp under tomcat.
After im Getting "Fatal error: Class 'Model' not found in E:\wamp\www\mycakeapp\cake\libs\model\app_model.php on line 38"
So, what is the solution for this problem can u help me.
Where can I get an official documentations on this Google analytics API .
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
[...] Google Analytics PHP Api (CakePHP Model): "Vote for this story on digg.com: Digg It! [...]