Git remote hates you
Posted on 17/11/09 by Felix Geisendörfer
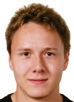
No, you didn't do anything wrong. Git sometimes is like your best friend who secretly hates you. Let's say you start a fresh new project:
mkdir new-project cd new-project git init touch README git add README git commit -m 'first commit' git remote add origin git@github.com:felixge/new-project.git git push origin master
So far so good. But - if like any self respecting geek, you juggle a million git repositories on your machine - you will soon have forgotten whether you started or cloned this particular repository. If you are unlucky that means you will run into this:
% git push fatal: The current branch master is not tracking anything.
Not helpful. "git pull" seems to try to make up for it by giving you way too much information:
% git pull You asked me to pull without telling me which branch you want to merge with, and 'branch.master.merge' in your configuration file does not tell me either. Please specify which branch you want to merge on the command line and try again (e.g. 'git pull <repository> <refspec>'). See git-pull(1) for details. If you often merge with the same branch, you may want to configure the following variables in your configuration file: branch.master.remote = <nickname> branch.master.merge = <remote-ref> remote.<nickname>.url = <url> remote.<nickname>.fetch = <refspec> See git-config(1) for details.
Ok, so what exactly do I have to do to fix this? Right, you ignore all those blobs in your repository making fun of you, and type:
git pull origin master
Using git and feeling like one has a certain overlap in the beginning. On your way to git enlightenment, or to the madhouse, you may eventually discover the fix:
git config branch.master.merge refs/heads/master git config branch.master.remote origin
And no, do not, not even for a second, assume you could skip "branch.master.remote". Git remote will be very clear about how much it hates you if you do:
% git pull You asked me to pull without telling me which branch you ... # The 2s delay made you suspicious, turn off wifi % git pull ssh: Could not resolve hostname github.com: nodename nor servname provided, or not known fatal: The remote end hung up unexpectedly
What in the name of the kernel? Git clearly knows the remote you are talking about, its merely teasing you, possibly corrupting your repo by turning some blobs into LOLcats. For added frustration, here is my output for "git push":
% git push Bus error
This is probably unique to my install. In case git does not hate you equally, you can try to complain about it in #git. That of course will only result in people telling you that you are being unreasonable. To a kernel hacker, the idea of git remote making some smart assumptions when adding the first remote to your fresh repository that only has a single branch, that is like talking healthcare reform with a right-wing hardliner.
Disclaimer: I love git, but some parts of it seem to purely back up the name. But relax. I'll save talking about git modules, tracking empty folders and checking out partial trees for another time ...
-- Felix Geisendörfer aka the_undefined