Simple ListsHelper for displaying nested ul / ol lists
Posted on 29/6/06 by Felix Geisendörfer
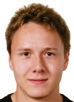
Deprecated post
The authors of this post have marked it as deprecated. This means the information displayed is most likely outdated, inaccurate, boring or a combination of all three.
Policy: We never delete deprecated posts, but they are not listed in our categories or show up in the search anymore.
Comments: You can continue to leave comments on this post, but please consult Google or our search first if you want to get an answer ; ).
Update: I opened a ticket for this functionality. See Ticket 1103.
Today I needed to display a simple ul list in one of my views, and was pretty surprised that the html helper didn't offer some kind of function for this. So I just went ahead and wrote my own little ListsHelper to compensate this lag for now. However, I'll open a ticket asking for this functionality in the html helper since I think it's pretty basic.
The code doesn't know any fancy ways to style the individual li's, so it's only really good for simple purposes, but here we go:
{
var $helpers = array('Html');
function ul($items, $htmlAttributes = null, $return = false)
{
$out ="<ul" . $this->Html->_parseAttributes($htmlAttributes) . ">\n";
$out = $out . $this->__createLiList($items);
$out = $out."</ul>\n";
return $this->output($out, $return);
}
function ol($items, $htmlAttributes = null, $return = false)
{
$out ="<ol" . $this->Html->_parseAttributes($htmlAttributes) . ">\n";
$out = $out . $this->__createLiList($items);
$out = $out."</ol>\n";
return $this->output($out, $return);
}
function __createLiList($items)
{
$out = "";
foreach ($items as $key => $val)
{
if (is_array($val))
{
$out = $out."<li>".$this->__createLiList($val)."</li>\n";
}
else
{
$out = $out."<li>".$val."</li>\n";
}
}
return $out;
}
}
Usage is pretty simple, just take an array and pass it to the function (after you've added the Lists helper to your var $helpers in controller):
--Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
Hi ad,
the reason my helper doesn't extend the HtmlHelper is pretty simple. I want the HtmlHelper to be accessible via $html. So when I would extend the ListsHelper from HtmlHelper I would end up with 2 HtmlHelpers, one of them in $lists (with ol and ul function) and the old one in $html. Seems like wasted memory to me. Now there are ways to extend CakePhp's CoreHelpers to do what you want and make them accessible with their standard names (like $html), and in fact I've done that before (search this blog for FlexiFix). But for if I want to share my code later on, only people with the same fix to the HtmlHelper could use my code with $html->ul() in it, all others would just go crazy trying to figure out why their $html Helper doesn't offer a function like this.
I hope this comment of mine made sense to you, I sometimes suck at explaining my OOP decisions : P.
[...] ThinkingPHP » Simple ListsHelper for displaying nested ul / ol lists A simple helper to manage ul’s (tags: cakephp helper) [...]
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
Thanks Felix, I also agree it is much needed - lists are fundamental. A quick question about the way you have extended the CakePHP code though.... I'm still trying to understand how Cake has been designed. I see you make use of the HTML helper by including it in the $helpers array. Is there a reason you don't use inheritance? (class ListsHelper extends HtmlHelper...)
I would have thought extending the HTML helper would be the way to go, giving you direct access to the (pseudo) private HtmlHelper methods such as _parseAttributes, but is there something in the design of Cake that recommends this "has a" relationship as opposed to "is a" inheritence ?